Book Recommendations for Developers
According to surveys, reading books in the world of development remains one of the strongest study options. Here are some recommendations.
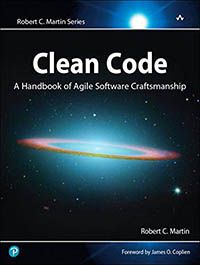
Clean Code: A Handbook of Agile Software Craftsmanship
This book by Robert C. Martin is essential for programmers looking to improve the quality of their code. The book provides principles and practices for writing clear, efficient, and maintainable code, highlighting the importance of professional ethics in software development. It is a valuable resource for both beginners and experienced professionals seeking to refine their skills and adopt a more disciplined approach to their work.
What you can learn
- Clean Code Principles: How to write code that is readable, maintainable, and efficient.
- Meaningful Names: The importance of choosing clear and descriptive names for variables, functions, and classes.
- Small, Focused Functions: How to structure functions to perform a single task and be easy to understand.
- Error Handling: Strategies for managing errors in a way that doesn't interfere with code logic.
- Code Formatting: Best practices for keeping code clean and consistent in appearance.
- Useful Comments: When and how to use comments to improve code understanding without cluttering it.
- Objects and Data Structures: Principles for designing objects that are easy to use and modify.
- Classes: How to design coherent classes with well-defined responsibilities.
- Unit Testing: The importance of automated testing to ensure code quality.
- Refactoring: Techniques to improve and simplify existing code without changing its behavior.
- Concurrency: Principles for correctly handling concurrency in applications, minimizing errors and complexity.
- Software Design Principles: Guidelines on applying design principles like SOLID to create more robust systems.
- Discipline and Professional Ethics: The importance of responsibility and ethics in software development, especially when working in teams and maintaining long-term projects.
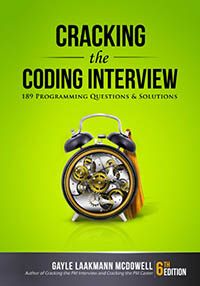
Cracking the Coding Interview
This book by Gayle Laakmann McDowell is an essential guide for any programmer aspiring to successfully pass technical interviews at leading tech companies. The book offers a combination of interview questions, detailed solutions, and practical advice that prepare candidates for the challenges they will face during the selection process. McDowell not only covers technical aspects but also strategies for presenting ideas clearly and handling pressure, making this book a valuable resource for those aiming to stand out in high-level interviews.
What you can learn
- Technical Interview Questions: A comprehensive collection of questions covering topics like algorithms, data structures, and system design.
- Detailed Solutions: Step-by-step explanations on how to solve the most common technical interview questions.
- Fundamental Concepts: Review of essential topics such as Big O, recursion, and graph theory.
- Problem-Solving Strategies: Methods for tackling and solving complex problems during an interview.
- Interview Preparation: Tips on how to mentally and emotionally prepare for technical interviews.
- Presenting Solutions: Techniques for effectively communicating your ideas and solutions during an interview.
- Behavioral Interviews: Guidance on how to approach behavioral and situational interview questions.
- Mock Interviews: Exercises to practice technical interviews in a simulated environment.
- System Design: Introduction to system design questions and problems presented in high-level interviews.
- Additional Resources: Recommendations for further resources and tools to enhance your technical skills.
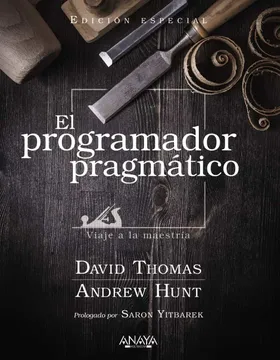
The Pragmatic Programmer
This book by Andrew Hunt and David Thomas is a fundamental read for any developer seeking to improve their approach and work philosophy in software development. It goes beyond coding techniques, covering a set of principles, best practices, and pragmatic approaches that can be applied to various situations throughout a programmer's career. With clear style and practical advice, Hunt and Thomas teach how to think and act as an agile and efficient programmer, making this book an indispensable read for those aiming to be more than just coders.
What you can learn
- Pragmatic Thinking: How to adopt a pragmatic mindset in software development, focused on flexibility and efficiency.
- Coding Best Practices: Techniques for writing clean, maintainable, and adaptable code.
- Automation: The importance of automating repetitive tasks to improve productivity.
- Project Management: Tips on how to effectively manage software projects, including planning and estimation.
- Refactoring: Strategies for continuously improving and refactoring existing code.
- Testing and Debugging: Pragmatic approaches to testing and debugging software to ensure its quality and functionality.
- Continuous Development: How to stay up-to-date with new technologies and continuously improve as a professional.
- Effective Communication: Techniques for communicating ideas clearly and efficiently with your team and clients.
- Tools and Techniques: Exploration of tools and techniques that can enhance a developer's workflow.
- Problem Prevention: How to anticipate and prevent common problems in software development before they arise.
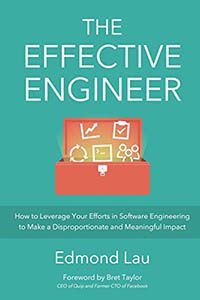
The Effective Engineer
This book by Edmond Lau is a practical guide designed to help software engineers maximize their impact and productivity in their careers. Lau presents a series of strategies and principles based on real experiences at some of the most successful tech companies. The book focuses on how engineers can make more efficient use of their time, make more effective technical decisions, and contribute significantly to their teams and projects. With an emphasis on prioritization and efficiency, "The Effective Engineer" is essential reading for any engineer who wants to advance their career and achieve greater impact.
What you can learn
- Maximizing Impact: Strategies for identifying and focusing on tasks that generate the greatest impact on your work and team.
- Effective Prioritization: How to prioritize projects and tasks to maximize productivity and avoid distractions.
- Task Automation: The importance of automating repetitive tasks to free up time for more valuable work.
- Workflow Optimization: Techniques for improving workflow and reducing wasted time and resources.
- Scalability: Principles for designing systems and processes that can efficiently scale as growth occurs.
- Measurement and Analysis: How to use metrics and analysis to guide technical decisions and measure progress.
- Effective Collaboration: Strategies for improving collaboration within the team and with other departments.
- Continuous Learning: Tips on how to stay up-to-date and continuously learn to remain effective.
- Communicating Ideas: Techniques for communicating ideas and technical decisions clearly and persuasively.
- Time Management: Strategies for effectively managing time, avoiding burnout, and maintaining high performance.
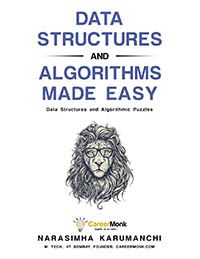
Data Structures and Algorithms Made Easy
This book by Narasimha Karumanchi is designed to simplify the learning of data structures, a fundamental topic for any programmer. Karumanchi provides a clear and concise explanation of data structures, accompanied by numerous examples and practical exercises. The book is particularly useful for those preparing for technical interviews, as it covers not only theoretical concepts but also shows how to apply them in real-world situations. With a focus on practice and problem-solving, "Data Structures Made Easy" is an excellent tool for students and professionals looking to master this essential topic.
What you can learn
- Basic Data Structures Concepts: Introduction to fundamental data structures like arrays, linked lists, stacks, and queues.
- Trees and Graphs: How to work with hierarchical and network structures such as binary trees, search trees, and graphs.
- Search and Sorting Algorithms: Explanation of essential algorithms like binary search, quicksort, and mergesort.
- Hash Tables: Understanding how hash tables work and their application in efficient searching.
- String Manipulation Algorithms: Techniques for handling and processing strings in programming.
- Algorithm Optimization: How to analyze and improve the efficiency of algorithms using Big O notation.
- Advanced Data Structures: Exploration of more complex structures like heaps, tries, and directed graphs.
- Practical Applications: Examples of how to use data structures in real-world programming problems and software development.
- Interview Preparation: Exercises and practice problems aimed at preparing for technical interviews in tech companies.
- Problem-Solving: Strategies for approaching and solving programming problems using data structures.
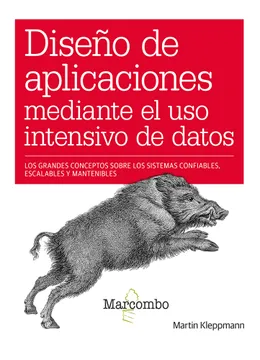
Designing Data-Intensive Applications: The Big Ideas Behind Reliable, Scalable, and Maintainable Systems
This book by Martin Kleppmann is a comprehensive guide that addresses the fundamental principles for designing systems that handle large volumes of data reliably, scalably, and maintainably. The book delves into key concepts behind distributed systems architecture, databases, and data management in modern applications. Kleppmann combines theory with practical examples to provide a clear understanding of complex topics like replication, partitioning, consistency, and fault tolerance. It is a must-read for software engineers and architects looking to build robust, future-proof systems.
What you can learn
- Distributed Systems: Fundamentals of designing and architecting distributed systems and how to manage them efficiently.
- Databases and Storage: Different database models (relational, NoSQL, NewSQL) and their appropriate use cases.
- Data Replication: Techniques for replicating data to improve system availability and reliability.
- Data Partitioning: Strategies for partitioning data and managing horizontal scaling in distributed applications.
- Consistency and Consensus: Concepts of consistency in distributed databases and consensus algorithms like Paxos and Raft.
- Fault Tolerance: Designing systems that can tolerate failures and continue to function effectively.
- Real-Time Data Processing: Techniques and architectures for processing and analyzing large volumes of data in real time.
- Batch Processing: How to design efficient systems for batch processing of large data sets.
- Data Streams: Implementation and management of data streams in real-time applications.
- Monitoring and Maintenance: Best practices for monitoring, debugging, and maintaining data-intensive applications.
- Scalability and Performance: Strategies for designing scalable systems that maintain high performance as they grow.
- Use Cases and Practical Examples: Analysis of real-world architectures and lessons learned from large-scale systems in the industry.